Contents
Called Functions
VL11: Ausgleichsrechnung
clear all; close all;
t = (0:0.01:3)';
y = 4 + cos(t) - 0.5*sin(t)+0.2*rand(size(t));
plot(t,y,'r.')
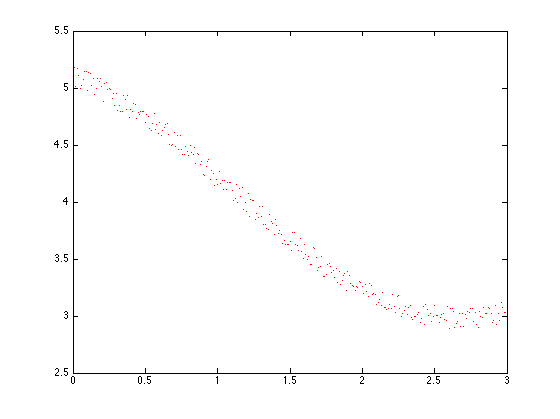
Ziel: Bestimme eine Kurve, die diese Daten gut approximiert.
1. Versuch: Polynominterpolation
close all; tt = 0:0.001:3; yy = polyinterp(t,y,tt); plot(t,y,'r.') hold on plot(tt,yy) axis([1 2 2 5]) hold off
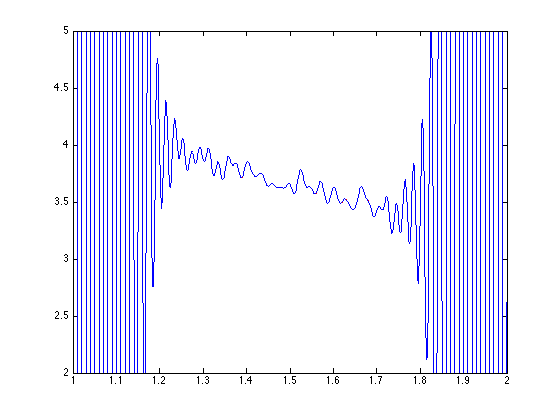
2. Versuch: Berechne Ausgleichsgrade
X1 = [ones(size(t)) t]; c1 = X1\y; f1 = @(t) [ones(size(t)) t]*c1; close all plot(t,y,'r.'); hold on ezplot(f1,[0,3])
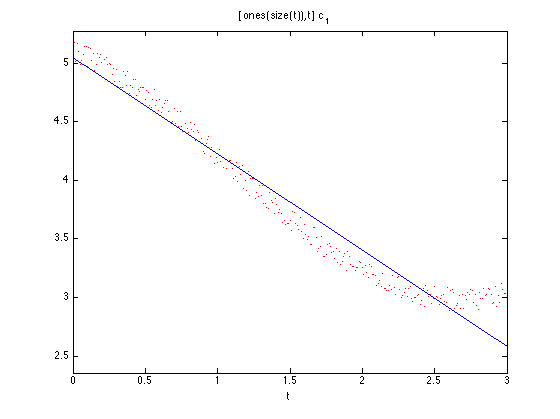
3. Versuch: Berechne Polynom
X2 = [ones(size(t)) t t.^2]; c2 = X2\y; f2 = @(t) [ones(size(t)) t t.^2]*c2; close all plot(t,y,'r.'); hold on ezplot(f2,[0,3])
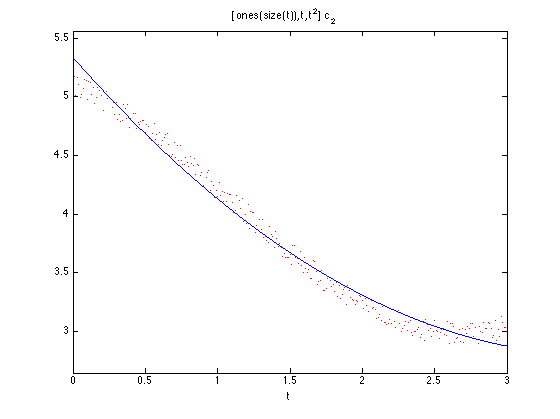
X2 = [ones(size(t)) t t.^2 t.^3]; c2 = X2\y; f2 = @(t) [ones(size(t)) t t.^2 t.^3]*c2; close all plot(t,y,'r.'); hold on ezplot(f2,[0,3])
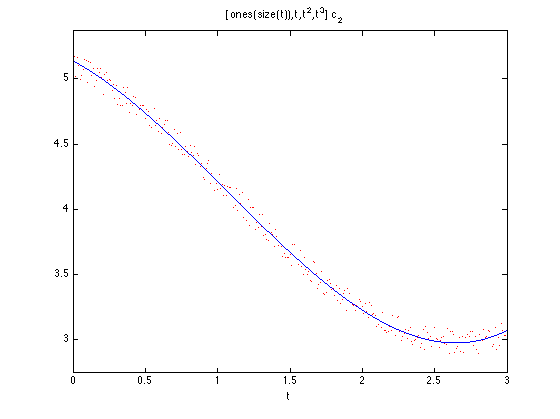
4. Versuch: Benutze die Basis 1, sin(t), cos(t):
X3 = [ones(size(t)) cos(t) sin(t)]; c3 = X3\y; f = @(t) [ones(size(t)) cos(t) sin(t)]*c3; close all plot(t,y,'r.'); hold on; ezplot(f,[0 3])
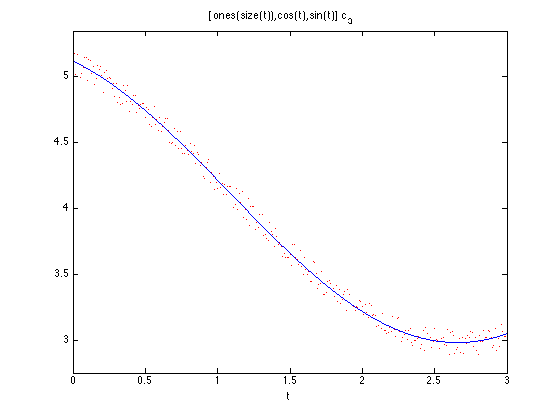
Verwende QR-Zerlegung
X4 = [ones(size(t)) cos(t) sin(t)]; [Q,R]=qr(X4,0); c4 = R\(Q'*y); f = @(t) [ones(size(t)) cos(t) sin(t)]*c4; close all; plot(t,y,'r.'); hold on; ezplot(f,[0,3])
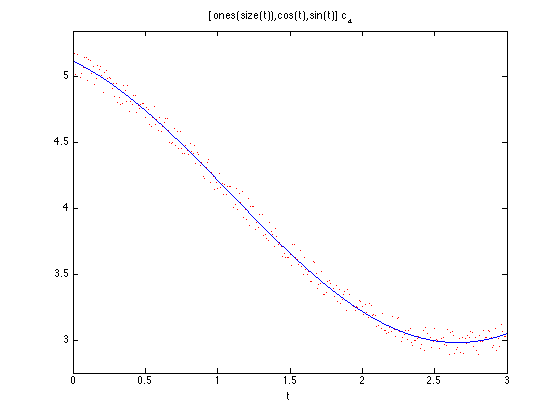
Veranschaulichung der Funktionsweise von qr(X,y)
t = (0:0.25:1)'; y = 4 + cos(t) - 0.5*sin(t)+0.2*rand(size(t)); X = [ones(size(t)) cos(t) sin(t)]; qrsteps(X,y)
A = 1.0000 1.0000 0 1.0000 0.9689 0.2474 1.0000 0.8776 0.4794 1.0000 0.7317 0.6816 1.0000 0.5403 0.8415 b = 5.1364 4.8537 4.6522 4.4952 4.1389 A = -2.2361 -1.8418 -1.0062 0 0.0907 -0.0635 0 -0.0006 0.1685 0 -0.1465 0.3707 0 -0.3379 0.5305 b = -10.4095 0.0497 -0.1518 -0.3087 -0.6650 A = -2.2361 -1.8418 -1.0062 0 -0.3793 0.6313 0 0 0.1676 0 0 0.1542 0 0 0.0311 b = -10.4095 -0.7238 -0.1508 -0.0676 -0.1089 A = -2.2361 -1.8418 -1.0062 0 -0.3793 0.6313 0 0 -0.2298 0 0 0 0 0 0 b = -10.4095 -0.7238 0.1701 0.0568 -0.0838 ans = -2.2361 -1.8418 -1.0062 0 -0.3793 0.6313 0 0 -0.2298 0 0 0 0 0 0